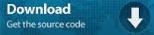
In this article i will explain
how to select and upload multiple
files and or images through
single Fileupload control in asp.net using both C# and VB languages with the
help of jQuery Multifile plugin.
Implementation: Let's create a simple webpage to demonstrate the concept.
Implementation: Let's create a simple webpage to demonstrate the concept.
- First of all create a
folder "Images" in the root of the project. All the uploaded
files will be stored in this folder.
- Next you need jQuery script files that
are required to upload
multiple files.
Download demo project for these files.
Asp.Net C# Section
Design Section:
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title>Upload Multiple Files or images</title>
<script
src="Scripts/jquery-1.11.1.min.js"
type="text/javascript"></script>
<script
src="Scripts/jquery.MultiFile.js"
type="text/javascript"></script>
</head>
<body>
<form
id="form1"
runat="server">
<div>
<fieldset
style="width:400px; margin-left:240px; margin-top:90px;">
<legend><strong>Upload Multiple Files or images Using Jquery</strong></legend>
<asp:FileUpload ID="FileUpload1" runat="server" class="multi" />
<br />
<asp:Button ID="btnUpload" runat="server" Text="Upload"
onclick="btnUpload_Click" />
<asp:Label ID="lblStatus" runat="server"></asp:Label>
</fieldset>
</div>
</form>
</body>
</html>
Asp.Net C# Code to upload multiple files/images
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Text;
using System.Drawing;
public partial class MultipleUpload_Csharp : System.Web.UI.Page
{
protected void btnUpload_Click(object sender, EventArgs e)
{
StringBuilder sb = new StringBuilder();
try
{
HttpFileCollection hfc = Request.Files;
for (int i = 0; i < hfc.Count; i++)
{
HttpPostedFile hpf = hfc[i];
if (hpf.ContentLength > 0)
{
hpf.SaveAs(Server.MapPath("Images") + "\\" + System.IO.Path.GetFileName(hpf.FileName));
sb.Append(String.Format("<br/><b>File Name: </b> {0}
<b>File Size:</b> {1} <b>File Type:</b> {2}
uploaded successfully", hpf.FileName, hpf.ContentLength,
hpf.ContentType));
}
}
lblStatus.ForeColor = Color.Green;
lblStatus.Text = sb.ToString();
}
catch (Exception ex)
{
lblStatus.ForeColor = Color.Red;
lblStatus.Text = "Error
occured: " +
ex.Message.ToString();
}
}
}
Asp.Net VB Code to upload multiple
images/files
Imports System.Drawing
Imports System.Text
Partial Class Multipleupload_VB
Inherits System.Web.UI.Page
Protected Sub btnUpload_Click(sender As Object, e As EventArgs) Handles btnUpload.Click
Dim sb As New StringBuilder()
Try
Dim hfc As HttpFileCollection = Request.Files
For i As Integer = 0 To hfc.Count - 1
Dim hpf As HttpPostedFile = hfc(i)
If hpf.ContentLength > 0 Then
hpf.SaveAs(Server.MapPath("Images") & "\" & System.IO.Path.GetFileName(hpf.FileName))
sb.Append([String].Format("<br/><b>File Name: </b> {0}
<b>File Size:</b> {1} <b>File Type:</b> {2}
uploaded successfully", hpf.FileName, hpf.ContentLength,
hpf.ContentType))
End If
Next
lblStatus.ForeColor = Color.Green
lblStatus.Text = sb.ToString()
Catch ex As Exception
lblStatus.ForeColor = Color.Red
lblStatus.Text = "Error
occured: " &
ex.Message.ToString()
End Try
End Sub
End Class
0 comments:
Post a Comment