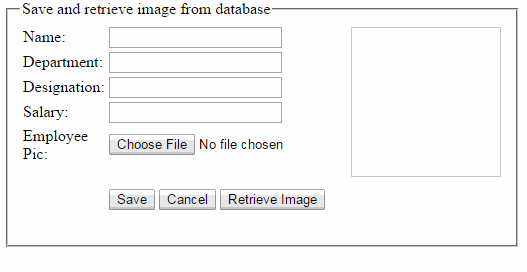
- Upload image through asp.net
fileupload control and store that image in folder.
- Save uploaded image path in Sql server database.
- Read/retrieve the image from the image path stored in
database and display on webpage.
In this demo application, I will save Employee details in database with image. Then Retrieve the details along with image and show on webpage.
Create a table "EmployeeDetail" in Sql
server Database as shown below:
CREATE TABLE [dbo].[EmployeeDetail](
[EmpId]
[int] NULL,
[Name]
[varchar](50) NULL,
[Department]
[varchar](50) NULL,
[Designation]
[varchar](50) NULL,
[salary]
[decimal](18, 2) NULL,
[PicName]
[varchar](100) NULL,
[PicPath]
[varchar](500) NULL
)
Then
create the following stored procedure in Sql server database
Stored
procedure to save Employee record in Database
CREATE PROCEDURE [dbo].[InsertEmpDetails_Sp]
@Name VARCHAR(100),
@dept VARCHAR(100),
@Desig VARCHAR(100),
@Salary DECIMAL(18,2),
@PicName VARCHAR(100)=NULL,
@PicPath VARCHAR(200)=NULL
AS
BEGIN
SET
NOCOUNT ON;
INSERT
INTO EmployeeDetail(Name,Department,Designation,salary,PicName,PicPath)
VALUES (@Name,@dept,@Desig,@Salary,@PicName,@PicPath)
END
Stored
procedure to fetch the Employee record from Database
CREATE PROCEDURE [dbo].[GetEmpDetails_Sp]
AS
BEGIN
SET
NOCOUNT ON;
SELECT
* FROM
EmployeeDetail
END
Create
Connection String In WebConfig file:
<connectionStrings>
<add name="Empcon" connectionString="Data
Source=Local host;Initial Catalog=Test;Integrated Security=True"/>
</connectionStrings>
Source Code:
Create a folder "Images" in the root directory of
project to upload Employee Images. The path will be saved into the database so
that we can read the image path and get the image from the folder to display.
Design
Section:
Create
the page as:
<fieldset style="width:400px;">
<legend>Save and
retrieve image from database</legend>
<table>
<tr><td>Name: </td><td><asp:TextBox ID="txtName"
runat="server"></asp:TextBox></td>
<td rowspan="8" valign="top"><asp:Image ID="Image1" runat="server"
Width="150px"
Height="150px"/>
<center><asp:Label ID="lbEmpPicName" runat="server" Text=""></asp:Label></center>
</td>
</tr>
<tr><td>Department: </td><td><asp:TextBox ID="txtDept"
runat="server"></asp:TextBox></td></tr>
<tr><td>Designation: </td><td><asp:TextBox ID="txtDesig"
runat="server"></asp:TextBox></td></tr>
<tr><td>Salary: </td><td><asp:TextBox ID="txtSalary"
runat="server"></asp:TextBox></td></tr>
<tr><td>Employee Pic:
</td><td>
<asp:FileUpload ID="flupEmpPic" runat="server" /></td></tr>
<tr><td> </td><td>
</td></tr>
<tr><td></td><td>
<asp:Button ID="btnSave" runat="server" Text="Save" onclick="btnSave_Click" />
<asp:Button ID="btnCancel" runat="server" onclick="btnCancel_Click"
Text="Cancel" />
<asp:Button ID="btnRetrieve"
runat="server"
onclick="btnRetrieve_Click"
Text="Retrieve Image" />
</td></tr>
<tr><td> </td><td>
<asp:Label ID="lblStatus" runat="server"></asp:Label>
</td></tr>
</table>
</fieldset>
Asp.Net C# Code to
store image and data and show on webpage
Write
the code as:
using
System;
using
System.Collections.Generic;
using
System.Linq;
using
System.Web;
using
System.Web.UI;
using
System.Web.UI.WebControls;
using
System.Data;
using
System.Data.SqlClient;
using
System.Configuration;
using
System.IO;
using
System.Drawing;
public partial class Upload : System.Web.UI.Page
{
SqlConnection
con = new SqlConnection(ConfigurationManager.ConnectionStrings["EmpCon"].ConnectionString);
protected void Page_Load(object
sender, EventArgs e)
{
}
protected void btnSave_Click(object
sender, EventArgs e)
{
string
fileName = string.Empty;
string
filePath = string.Empty;
string
getPath = string.Empty;
string
pathToStore = string.Empty;
string
finalPathToStore = string.Empty;
SqlCommand
cmd = new SqlCommand("InsertEmpDetails_Sp", con);
cmd.CommandType = CommandType.StoredProcedure;
cmd.Parameters.AddWithValue("@Name", txtName.Text.Trim());
cmd.Parameters.AddWithValue("@Dept", txtDept.Text.Trim());
cmd.Parameters.AddWithValue("@Desig", txtDesig.Text.Trim());
cmd.Parameters.AddWithValue("@Salary", Convert.ToDecimal(txtSalary.Text));
try
{
if
(flupEmpPic.HasFile)
{
fileName = flupEmpPic.FileName;
filePath = Server.MapPath("Images/" + System.Guid.NewGuid() + fileName);
flupEmpPic.SaveAs(filePath);
cmd.Parameters.AddWithValue("@PicName", fileName);
int getPos =
filePath.LastIndexOf("\\");
int
len = filePath.Length;
getPath =
filePath.Substring(getPos, len - getPos);
pathToStore = getPath.Remove(0,
1);
finalPathToStore = "~/Images/" + pathToStore;
cmd.Parameters.AddWithValue("@PicPath", finalPathToStore);
}
con.Open();
cmd.ExecuteNonQuery();
lblStatus.Text = "Employee Record saved successfully";
lblStatus.ForeColor =
System.Drawing.Color.Green;
ClearControls();
}
catch (Exception ex)
{
lblStatus.Text = "Employee Record could not be saved";
lblStatus.ForeColor =
System.Drawing.Color.Red;
}
finally
{
con.Close();
cmd.Dispose();
fileName = null;
filePath = null;
getPath = null;
pathToStore = null;
finalPathToStore = null;
}
}
protected void btnCancel_Click(object
sender, EventArgs e)
{
ClearControls();
lblStatus.Text = string.Empty;
}
private void ClearControls()
{
txtDept.Text = string.Empty;
txtName.Text = string.Empty;
txtSalary.Text = string.Empty;
txtDesig.Text = string.Empty;
txtName.Focus();
}
protected void btnRetrieve_Click(object
sender, EventArgs e)
{
SqlDataReader dr = null;
SqlCommand
cmd = new SqlCommand("GetEmpDetails_Sp", con);
cmd.CommandType = CommandType.StoredProcedure;
con.Open();
try
{
dr = cmd.ExecuteReader();
if
(dr.HasRows)
{
dr.Read();
txtName.Text = Convert.ToString(dr["Name"]);
txtDept.Text = Convert.ToString(dr["Department"]);
txtDesig.Text = Convert.ToString(dr["Designation"]);
txtSalary.Text = Convert.ToString(dr["Salary"]);
if
(!string.IsNullOrEmpty(Convert.ToString(dr["PicPath"])))
{
Image1.ImageUrl =Convert.ToString(dr["PicPath"]);
lbEmpPicName.Text = Convert.ToString(dr["PicName"]);
}
lblStatus.Text = "Employee detail record retrieved successfully";
lblStatus.ForeColor = Color.Green;
}
}
catch (Exception)
{
lblStatus.Text = "Employee record could not be retrieved";
lblStatus.ForeColor = Color.Red;
}
finally
{
dr.Dispose();
con.Close();
cmd.Dispose();
}
}
}
Asp.Net VB Section:
Design the page as:
<fieldset style="width:400px;">
<legend>Save and retrieve image from database</legend>
<table>
<tr><td>Name: </td><td><asp:TextBox ID="txtName" runat="server"></asp:TextBox></td>
<td rowspan="8" valign="top"><asp:Image ID="Image1" runat="server"
Width="150px"
Height="150px"/>
<center><asp:Label ID="lbEmpPicName"
runat="server"
Text=""></asp:Label></center>
</td>
</tr>
<tr><td>Department: </td><td><asp:TextBox ID="txtDept" runat="server"></asp:TextBox></td></tr>
<tr><td>Designation: </td><td><asp:TextBox ID="txtDesig" runat="server"></asp:TextBox></td></tr>
<tr><td>Salary: </td><td><asp:TextBox ID="txtSalary" runat="server"></asp:TextBox></td></tr>
<tr><td>Employee Pic: </td><td>
<asp:FileUpload ID="flupEmpPic" runat="server" /></td></tr>
<tr><td> </td><td>
</td></tr>
<tr><td></td><td>
<asp:Button ID="btnSave" runat="server" Text="Save" />
<asp:Button ID="btnCancel" runat="server"
Text="Cancel"
/>
<asp:Button ID="btnRetrieve"
runat="server"
Text="Retrieve Image" />
</td></tr>
<tr><td> </td><td>
<asp:Label ID="lblStatus" runat="server"></asp:Label>
</td></tr>
</table>
</fieldset>
Asp.Net (VB.NET) Code to
store image and data and show in on webpage
Write the code as:
Imports System.Data
Imports System.Data.SqlClient
Imports System.Configuration
Imports System.IO
Imports System.Drawing
Partial Public Class
Upload
Inherits System.Web.UI.Page
Dim con As
SqlConnection = New
SqlConnection(ConfigurationManager.ConnectionStrings("EmpCon").ConnectionString)
protected void Page_Load(Object sender,
EventArgs e)
{
}
protected void btnSave_Click(Object
sender, EventArgs e)
{
Dim fileName As
String = String.Empty
Dim filePath As
String = String.Empty
Dim getPath As
String = String.Empty
Dim pathToStore As
String = String.Empty
Dim finalPathToStore As String = String.Empty
Dim cmd As
SqlCommand = New
SqlCommand("InsertEmpDetails_Sp",
con)
cmd.CommandType = CommandType.StoredProcedure
cmd.Parameters.AddWithValue("@Name", txtName.Text.Trim())
cmd.Parameters.AddWithValue("@Dept", txtDept.Text.Trim())
cmd.Parameters.AddWithValue("@Desig", txtDesig.Text.Trim())
cmd.Parameters.AddWithValue("@Salary",
Convert.ToDecimal(txtSalary.Text))
Try
if
(flupEmpPic.HasFile)
{
fileName = flupEmpPic.FileName
filePath =
Server.MapPath("Images/" + System.Guid.NewGuid() + fileName)
flupEmpPic.SaveAs(filePath)
cmd.Parameters.AddWithValue("@PicName", fileName)
Dim getPos As
Integer = filePath.LastIndexOf("\\")
Dim len As
Integer = filePath.Length
getPath =
filePath.Substring(getPos, len - getPos)
pathToStore = getPath.Remove(0,
1)
finalPathToStore =
"~/Images/" + pathToStore
cmd.Parameters.AddWithValue("@PicPath", finalPathToStore)
}
con.Open()
cmd.ExecuteNonQuery()
lblStatus.Text = "Employee
Record saved successfully"
lblStatus.ForeColor =
System.Drawing.Color.Green
ClearControls()
Catch
ex As Exception
lblStatus.Text =
"Employee Record could not be saved"
lblStatus.ForeColor =
System.Drawing.Color.Red
Finally
con.Close()
cmd.Dispose()
fileName = Nothing
filePath = Nothing
getPath = Nothing
pathToStore = Nothing
finalPathToStore = Nothing
End Try
}
protected void btnCancel_Click(Object
sender, EventArgs e)
{
ClearControls()
lblStatus.Text = String.Empty
}
private void ClearControls()
{
txtDept.Text = String.Empty
txtName.Text = String.Empty
txtSalary.Text = String.Empty
txtDesig.Text = String.Empty
txtName.Focus()
}
protected void btnRetrieve_Click(Object
sender, EventArgs e)
{
Dim dr As
SqlDataReader = Nothing
Dim cmd As
SqlCommand = New
SqlCommand("GetEmpDetails_Sp",
con)
cmd.CommandType =
CommandType.StoredProcedure
con.Open()
Try
dr = cmd.ExecuteReader()
if
(dr.HasRows)
{
dr.Read()
txtName.Text =
Convert.ToString(dr("Name"))
txtDept.Text =
Convert.ToString(dr("Department"))
txtDesig.Text =
Convert.ToString(dr("Designation"))
txtSalary.Text =
Convert.ToString(dr("Salary"))
if
(Not String.IsNullOrEmpty(Convert.ToString(dr("PicPath"))))
{
Image1.ImageUrl
=Convert.ToString(dr("PicPath"))
lbEmpPicName.Text =
Convert.ToString(dr("PicName"))
}
lblStatus.Text = "Employee
detail record retrieved successfully"
lblStatus.ForeColor =
Color.Green
}
Catch
lblStatus.Text = "Employee record
could not be retrieved"
lblStatus.ForeColor = Color.Red
Finally
dr.Dispose()
con.Close()
cmd.Dispose()
End Try
}
End Class
0 comments:
Post a Comment