Introduction:
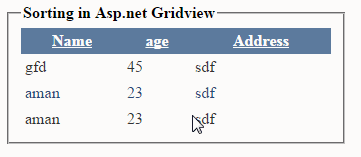
In this article i am going to explain how to enable
sorting in asp.net GridView records in ascending or descending order by
clicking on GridView's column header in Asp.net both using C# and VB language.
Steps to Follow:
1.
set the AllowSorting property to true
2.
In template field set SortExpression property to
column name in database. After setting
these properties the Columns in Gridview's header will turn into clickable links.
3.
After setting all the properties we need to do
some code in asp.net. We use viewstate to maintain sorting.
Implementation: Let's create a demo website to demonstrate the concept.
Create a
database “Test”, Then create one table i.e. Emp_Personal
Column
Name
|
Data
Type
|
EmpPer_Id
|
Int(Primary Key. So set is
identity=true)
|
EmpName
|
varchar(100)
|
Age
|
Int
|
Address
|
varchar(500)
|
create the connection string in Webconfig
as:
<connectionStrings>
<add name="Empcon" connectionString="Data Source=localhost;Initial
Catalog=test;IntegratedSecurity=True"/>
</connectionStrings>
Source Code:
DesignSection:
<div>
<fieldset style="width:310px;">
<legend><strong>Sorting in Asp.net Gridview</strong></legend>
<asp:GridView ID="grdEmpDetails"
runat="server"
Width="100%"
AutoGenerateColumns="False" AllowSorting="True" CellPadding="4"
ForeColor="#333333" GridLines="None" onsorting="grdEmpDetails_Sorting">
<AlternatingRowStyle BackColor="White" ForeColor="#284775" />
<Columns>
<asp:TemplateField HeaderText="Name"
SortExpression="EmpName">
<ItemTemplate>
<asp:Label ID="lblEmpName"
Text='<%#Eval("EmpName")%>' runat="server"
/>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="age"
SortExpression="age">
<ItemTemplate>
<asp:Label ID="lblAge" Text='<%#Eval("age")%>' runat="server"
/>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Address"
SortExpression="Address">
<ItemTemplate>
<asp:Label ID="lblAddress"
Text='<%#Eval("Address")%>' runat="server"
/>
</ItemTemplate>
</asp:TemplateField>
</Columns>
<EditRowStyle BackColor="#999999" />
<FooterStyle BackColor="#5D7B9D" Font-Bold="True" ForeColor="White" />
<HeaderStyle BackColor="#5D7B9D" Font-Bold="True" ForeColor="White" />
<PagerStyle BackColor="#284775" ForeColor="White" HorizontalAlign="Center" />
<RowStyle BackColor="#F7F6F3" ForeColor="#333333" />
<SelectedRowStyle BackColor="#E2DED6" Font-Bold="True" ForeColor="#333333" />
<SortedAscendingCellStyle BackColor="#E9E7E2" />
<SortedAscendingHeaderStyle BackColor="#506C8C" />
<SortedDescendingCellStyle BackColor="#FFFDF8" />
<SortedDescendingHeaderStyle BackColor="#6F8DAE" />
</asp:GridView>
</fieldset>
</div>
C#.Net code to
implement sorting in GridView
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
public partial class sortgrid :
System.Web.UI.Page
{
DataTable dt = new
DataTable();
protected void
Page_Load(object sender, EventArgs e)
{
if (!Page.IsPostBack)
{
ViewState["sortOrder"] = "";
BindGridView("", "");
}
}
private void
BindGridView(string sortExp, string sortDir)
{
SqlConnection con = new
SqlConnection(ConfigurationManager.ConnectionStrings["Empcon"].ConnectionString);
SqlCommand cmd = new
SqlCommand("select
* from Emp_Personal", con);
SqlDataAdapter adp = new
SqlDataAdapter(cmd);
adp.Fill(dt);
if (dt.Rows.Count > 0)
{
DataView dv = new DataView();
dv = dt.DefaultView;
if (sortExp != string.Empty)
{
dv.Sort = string.Format("{0} {1}", sortExp, sortDir);
}
grdEmpDetails.DataSource = dv;
grdEmpDetails.DataBind();
}
}
public string
sortOrder
{
get
{
if (ViewState["sortOrder"].ToString()
== "desc")
{
ViewState["sortOrder"] = "asc";
}
else
{
ViewState["sortOrder"] = "desc";
}
return ViewState["sortOrder"].ToString();
}
set
{
ViewState["sortOrder"] = value;
}
}
protected void
grdEmpDetails_Sorting(object sender, GridViewSortEventArgs e)
{
BindGridView(e.SortExpression, sortOrder);
}
}
VB.Net code to
implement sorting in GridView
Design the page as shown in HTML Source as mentioned above but
remove
onsorting="grdEmpDetails_Sorting" from the GridView source code.
Include
the following namespaces :
Imports System.Data
Imports System.Data.SqlClient
Imports System.Configuration
Then
write the code as:
Dim dt As New DataTable
Protected Sub Page_Load(sender As Object, e As System.EventArgs) Handles Me.Load
If Not Page.IsPostBack Then
ViewState("sortOrder") = ""
BindGridView("", "")
End If
End Sub
Private Sub BindGridView(sortExp As String, sortDir As String)
Dim con As New SqlConnection(ConfigurationManager.ConnectionStrings("Empcon").ConnectionString)
Dim cmd As New SqlCommand("select
* from EmpDetails", con)
Dim adp As New SqlDataAdapter(cmd)
adp.Fill(dt)
If dt.Rows.Count > 0 Then
Dim dv As New DataView()
dv = dt.DefaultView
If sortExp <> String.Empty Then
dv.Sort = String.Format("{0}
{1}", sortExp, sortDir)
End If
grdBookDetails.DataSource = dv
grdBookDetails.DataBind()
End If
End Sub
Public Property sortOrder() As String
Get
If ViewState("sortOrder").ToString()
= "desc" Then
ViewState("sortOrder") = "asc"
Else
ViewState("sortOrder") = "desc"
End If
Return ViewState("sortOrder").ToString()
End Get
Set(value As String)
ViewState("sortOrder") = value
End Set
End Property
Protected Sub grdEmpDetails
_Sorting(sender As Object, e AsSystem.Web.UI.WebControls.GridViewSortEventArgs) Handles grdEmpDetails.Sorting
BindGridView(e.SortExpression, sortOrder)
End Sub
0 comments:
Post a Comment