Introduction:
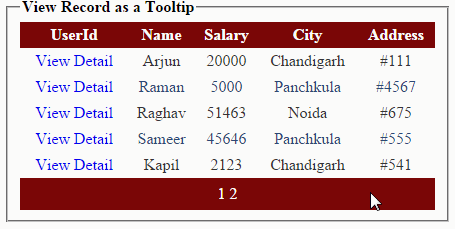
Steps To follow to
implement the tooltip on mousehover:
1. Download the tooltip jquery. To
download ClickHere.
2. Get
data to using stored procedure from database for table employee.
Create proc [dbo].[ BindEmp]
AS
BEGIN
select * from dbo.employee
end
3. Add a webpage and add following
script and controls:
Add the script and
style in head of page:
<script src="http://code.jquery.com/jquery-1.8.2.js" type="text/javascript"></script>
<script src="Scripts/jquery.tooltip.min.js" type="text/javascript"></script>
<script type="text/javascript">
function InitializeToolTip() {
$(".grdEmp").tooltip({
track: true,
delay: 0,
showURL: false,
fade: 100,
bodyHandler: function () {
return $($(this).next().html());
},
showURL: false
});
}
</script>
<script type="text/javascript">
$(function () {
InitializeToolTip();
})
</script>
<style type="text/css">
a {text-decoration: none;}
a : hover {color: #fffff;}
#tooltip {
position: absolute;
z-index: 3000;
border: 1px solid #111;
background-color: #CCCCCC;
padding: 5px;
opacity: 0.95; }
#tooltip h3, #tooltip div
{ margin: 0; }
</style>
Full Source Code:
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<script src="http://code.jquery.com/jquery-1.8.2.js"
type="text/javascript"></script>
<script src="Scripts/jquery.tooltip.min.js" type="text/javascript"></script>
<script type="text/javascript">
function
InitializeToolTip() {
$(".grdEmp").tooltip({
track: true,
delay: 0,
showURL: false,
fade: 100,
bodyHandler: function () {
return
$($(this).next().html());
},
showURL: false
});
}
</script>
<script type="text/javascript">
$(function
() {
InitializeToolTip();
})
</script>
<style type="text/css">
a {text-decoration: none;}
a : hover {color: #fffff;}
#tooltip {
position:
absolute;
z-index:
3000;
border:
1px solid #111;
background-color:
#CCCCCC;
padding:
5px;
opacity:
0.95;
}
#tooltip
h3, #tooltip
div
{
margin: 0; }
</style>
</head>
<body>
<form id="form1" runat="server">
<div>
<fieldset style="width:415px;">
<legend> <strong>View Record as a Tooltip</strong></legend>
<asp:GridView ID="grdEmp" runat="server" AllowSorting="True" EmptyDataText="No records found"
CssClass="rowHover" RowStyle-CssClass="rowHover" ShowHeader="true"
AutoGenerateColumns="False"
AllowPaging="True"
PageSize="5"
CellPadding="4" ForeColor="#333333"
GridLines="None" Width="100%">
<AlternatingRowStyle BackColor="White" ForeColor="#284775" />
<Columns>
<asp:TemplateField HeaderText="UserId">
<ItemStyle Width="100px"
HorizontalAlign="Center"
/>
<ItemTemplate>
<a href="#"
class="grdEmp">View
Detail</a>
<div id="tooltip"
style="display: none;">
<table>
<tr>
<td style="white-space:
nowrap;"><b>Name:</b> </td>
<td><%# Eval("Name")%></td>
</tr>
<tr>
<td style="white-space:
nowrap;"><b>Salary:</b> </td>
<td><%# Eval("salary")%></td>
</tr>
<tr>
<td style="white-space:
nowrap;"><b>Address:</b> </td>
<td><%# Eval("Address")%></td>
</tr>
</table>
</div>
</ItemTemplate>
</asp:TemplateField>
<asp:BoundField HeaderText="Name" DataField="Name" ItemStyle-HorizontalAlign="Center" />
<asp:BoundField HeaderText="Salary" DataField="Salary" ItemStyle-HorizontalAlign="Center" />
<asp:BoundField HeaderText="City" DataField="City" ItemStyle-HorizontalAlign="Center" />
<asp:BoundField HeaderText="Address" DataField="Address" ItemStyle-HorizontalAlign="Center" />
</Columns>
<EditRowStyle BackColor="#999999" />
<FooterStyle BackColor="#ffffff" Font-Bold="True" ForeColor="White" />
<HeaderStyle BackColor="#7B0606" Font-Bold="True" ForeColor="White" />
<PagerStyle BackColor="#7B0606" ForeColor="White" HorizontalAlign="Center" />
<RowStyle CssClass="rowHover"
BackColor="#F7F6F3"
ForeColor="#333333"></RowStyle>
<SelectedRowStyle BackColor="#E2DED6" Font-Bold="True" ForeColor="#333333" />
<SortedAscendingCellStyle BackColor="#E9E7E2" />
<SortedAscendingHeaderStyle BackColor="#506C8C" />
<SortedDescendingCellStyle BackColor="#FFFDF8" />
<SortedDescendingHeaderStyle BackColor="#6F8DAE" />
<EmptyDataRowStyle Width
= "550px"
ForeColor="Red"
Font-Bold="true"
HorizontalAlign
= "Center"/>
</asp:GridView>
</fieldset>
</div>
</form>
</body>
</html>
Write the code in .aspx.cs file:
using System.Data;
using System.Data.SqlClient;
using System.Configuration
SqlConnection con = newSqlConnection(ConfigurationManager.ConnectionStrings["EmpCon"].ToString());
protected void Page_Load(object sender, EventArgs e)
{
if(!IsPostBack)
{
Bindemp();
}
}
public void Bindemp()
{
SqlCommand cmd = new SqlCommand("BindEmp", con);
con.Open();
SqlDataAdapter adp = new SqlDataAdapter(cmd);
DataTable dt = new DataTable();
adp.Fill(dt);
grdemp.DataSource = dt;
grdemp.DataBind();
con.Close();
}
In VB:
Imports System.Data
Imports System.Data.SqlClient
Imports System.Configuration
Private con As NewSqlConnection(ConfigurationManager.ConnectionStrings("Empcon").ToString())
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
If Not IsPostBack Then
Bindemp()
End If
End Sub
Public Sub Bindemp()
Dim cmd As New SqlCommand("BindEmp", con)
con.Open()
Dim adp As New SqlDataAdapter(cmd)
Dim dt As New DataTable()
adp.Fill(dt)
grdemp.DataSource = dt
grdemp.DataBind()
con.Close()
End Sub
After doing all the
tasks build the application and run to check the result.
0 comments:
Post a Comment