Introduction:
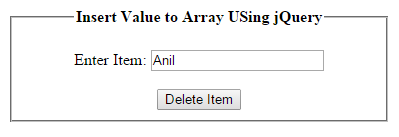
There are two methods in jquery with the help of that we can
delete items from array.
Using splice or grep properties
in Jquery we can delete or remove specific item / element from array list.
Grep() Method to delete Items
from array:
<script type="text/javascript">
$(function () {
var
arr = ["Anil", "Ashish", "Ankit",
"Anish"];
$('#btnDelete').click(function () {
var
str = $('#txtItem').val();
arr
= $.grep(arr, function (value) {
return value
!= str;
});
for (var i = 0; i < arr.length; i++) {
alert(arr[i])
}
});
})
</script>
Splice() Method to delete Items from
array:
<script type="text/javascript">
$(function () {
var
arr = ["Anil", "Ashish", "Ankit",
"Anish"];
$('#btnDelete').click(function () {
var
str = $('#txtItem').val();
for (var i = 0; i < arr.length; i++) {
if (arr[i] == str) {
arr.splice(i, 1);
}
}
for (var i = 0; i < arr.length; i++) {
alert(arr[i])
}
});
})
</script>
Full Source Code For sample
Application:
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<title>jquery delete items from array</title>
<script type="text/javascript"
src="http://code.jquery.com/jquery-1.8.2.js"></script>
<script type="text/javascript">
$(function
() {
var arr
= ["Anil", "Ashish",
"Ankit", "Anish"];
$('#btnDelete').click(function () {
var
str = $('#txtItem').val();
arr = $.grep(arr, function (value) {
return
value != str;
});
for
(var i = 0; i < arr.length; i++) {
alert(arr[i])
}
});
})
</script>
</head>
<body>
<center>
<fieldset style="width:350px">
<legend><strong>Delete
item From Array Using jQuery</strong></legend>
<div>
<br />
Enter Item: <input type="text" id="txtItem"
value="Anil"
/><br /><br />
<input type="button"
id="btnDelete"
value="Delete
Item" />
</div></fieldset></center>
</body>
</html>
0 comments:
Post a Comment