In this article I will explain how
to implement stylish jQuery form validation in
asp.net with example. If any input field is empty or data is not valid in that
field then a stylish message box will pop up with the appropriate message as
shown in image.
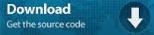
Whenever we creates any user input form like registration form or contact us form etc then it is required to implement validation on the fields to ensure that only valid data can be submitted to server for processing. Suppose in case of Age, only integer value should be entered. Password should be 6 characters long.
To validate data we can use ASP.NET validation. It can be done by using JavaScript and Jquery.
In this example, Validation rules implemented on each field is explained as:
First Name: Can’t be left blank.
Email Id: Can’t be left blank and should
be in valid email format.
Password: Can’t be left blank and minimum 6 digits or characters are required.
Confirm Password: Can’t be left blank and minimum 6 digits or characters are required and must match with the Password field.
Age: Can’t be left blank and should be digits and minimum age can be entered 18 and maximum age 25 can be entered.
Password: Can’t be left blank and minimum 6 digits or characters are required.
Confirm Password: Can’t be left blank and minimum 6 digits or characters are required and must match with the Password field.
Age: Can’t be left blank and should be digits and minimum age can be entered 18 and maximum age 25 can be entered.
Mobile No.: Can’t be left blank and
should be digits and 10 digits long.
DOB: Can’t be left blank and should
be in valid date format (YYY-MM-DD) format
Website: Can’t be left blank and
should be in valid URL format e.g. http://www.webcodeexpert.com
City: You must select the city.
Zip: Can’t be left blank and
should be digits and at least 6 digits long.
I agree to terms and
conditions: This
checkbox must be checked.
Let's create a Jquery validation form using jQuery. I have tried to cover
all type of input fields like textbox, dropdownlist or checkbox etc.
Follow the following steps:
- Download the sample project
attached with this article because "js" folder having required
jQuery files and the "css" folder having style sheets is
necessary for this implementation.
- Now create a new website and paste the "js" and
"css" in the root directory and then add a page
"default.aspx" in the website. It will look like as shown in the
image below:
In the
<Head> Section of design page
(default.aspx) link the style sheets and the required jquery files as:
<link href="css/template.css" rel="stylesheet" type="text/css" />
<link href="css/validationEngine.jquery.css" rel="stylesheet" type="text/css" />
<script src="js/jquery-1.8.2.min.js" type="text/javascript"></script>
<script src="js/jquery.validationEngine-en.js" type="text/javascript" charset="utf-8"></script>
<script src="js/jquery.validationEngine.js" type="text/javascript" charset="utf-8"></script>
<script type="text/javascript">
jQuery(document).ready(function() {
jQuery("#form1").validationEngine();
});
</script>
<noscript>
<h4 style="color: #FF3300;">
Javascript is not currently enabled in your browser.<br /> You must <ahref="http://support.google.com/bin/answer.py?hl=en&answer=23852" target="_blank" >enable
Javascript</a> to run
this web page correctly.
</h4>
</noscript>
</head>
I have
used <noscript> tag in the <Head> section. This is useful in the
case if the JavaScript is disabled on the browser running the website. This
<noscript> tag executes only if the javaScript is disabled in the
browser.
Javascript
should be enable if you want to run jquery on browser.
Now in the
<Body> tag design the registration
form as:
<form id="form1" runat="server">
<fieldset style="width:380px;">
<legend style="font-size:18px;"><strong>jQuery
Validation Form</strong></legend>
<table>
<tr>
<td colspan="2">
<div>
<table cellpadding="0"
cellspacing="10"
style=" background-color:White">
<tr>
<td colspan="2">All
the fields marked with (<span class="starMark">*</span>) are
mandatory fields</td>
</tr>
<tr>
<td>Name: <span class="starMark">*</span></td>
<td><asp:TextBox ID="txtfname" runat="server" CssClass="validate[required]"/></td>
</tr>
<tr >
<td>Email Id: <span class="starMark">*</span></td>
<td><asp:TextBox ID="txtemail"
runat="server" CssClass="validate[required,custom[email]]" />
</td>
</tr>
<tr >
<td>Password: <span class="starMark">*</span></td>
<td><asp:TextBox ID="txtPwd" runat="server"
TextMode="Password" CssClass="validate[required,minSize[6],maxSize[10]]"
/>
</td>
</tr>
<tr >
<td>Confirm password:
<span class="starMark">*</span></td>
<td><asp:TextBox ID="txtConfirmPwd"
runat="server"
TextMode="Password" CssClass="validate[required,minSize[6],maxSize[10],equals[txtPwd]]"
/>
</td>
</tr>
<tr>
<td>Age: <span class="starMark">*</span></td>
<td><asp:TextBox ID="txtAge" runat="server" CssClass="validate[required,custom[integer],min[18],max[25]]
"/></td>
</tr>
<tr>
<td>Mobile No.: <span class="starMark">*</span></td>
<td><asp:TextBox ID="txtMobile" runat="server" CssClass="validate[required,custom[integer],minSize[10],maxSize[10]]
"/></td>
</tr>
<tr>
<td>Date of Birth:<span class="starMark">*</span></td>
<td><asp:TextBox ID="txtDob" runat="server" CssClass="validate[required,custom[date],past[now]]
"/></td>
</tr>
<tr >
<td>Website Url: <span class="starMark">*</span></td>
<td><asp:TextBox ID="txtWebUrl"
runat="server"
CssClass="validate[required,custom[url]]
text-input" />
</td>
</tr>
<tr>
<td valign="top">Address:
</td>
<td>
<asp:TextBox ID="txtaddress"
runat="server"
TextMode="MultiLine"
Rows="4"
Columns="25"/></td>
</tr>
<tr>
<td>City: <span class="starMark">*</span></td>
<td>
<asp:DropDownList ID="ddlCity"
runat="server"
CssClass="validate[required]
radio">
<asp:ListItem value="">Choose
City</asp:ListItem>
<asp:ListItem Value="CH">Chandigarh</asp:ListItem>
<asp:ListItem value="PT">Patiala</asp:ListItem>
<asp:ListItem value="PK">Panchkula</asp:ListItem>
<asp:ListItem value="AB">Ambala</asp:ListItem>
</asp:DropDownList>
</td>
</tr>
<tr>
<td>Zip: <span class="starMark">*</span></td>
<td>
<asp:TextBox ID="txtZip" runat="server"
CssClass="validate[required,minSize[6],maxSize[6],custom[onlyNumberSp]]
text-input"/>
</td>
</tr>
<tr>
<td colspan="2"> </td>
</tr>
<tr>
<td colspan="2"><input class="validate[required] checkbox" type="checkbox"
id="agree"
name="agree"/>
I Agree to terms and Conditions <span class="starMark">*</span>
</td>
</tr>
<tr>
<td></td>
<td>
<asp:Button ID="btnSubmit"
runat="server"
Text="Submit"
onclick="btnSubmit_Click"
/>
</td>
</tr>
</table>
</div>
</td>
</tr>
</table>
</fieldset>
</form>
You can customize the validation rules as per your
requirement by making changes in "jquery.validationEngine-en.js"
contained in the "js" folder.
0 comments:
Post a Comment