Introduction:
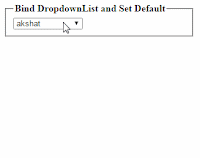
Steps to follow:
1. Add drop down list to webpage.
2.
Now get data from database using sql
query in code behind.
3.
Bind dropdown list with database i.e.
set DataTextField and DatValueField of the dropdown list.
4.
The column value set as DataTextField
will be visible to user.
5.
The column value set as DatValueField
will not be visible to anyone. This is used to identify Dropdown items uniquely.
6. No add default value at index value 0 of
the dropdown.
Implementation:
Create a database i.e.
“Test”. Then create a table
“Student_Info”.
Column Name
|
Datatype
|
Student_id
|
Int(Primary Key. So set Is
Identity=True)
|
Student_Name
|
Varchar(500)
|
Age
|
int
|
Class
|
Varchar(50)
|
Now
insert some data in this table using “insert” command.
Use Stored Procedure:
Create proc [dbo].[Fill_Dataset]
as
begin
select * from student_info
end
Create
Connection: Now create connection in
webconfig file as given below.
<connectionStrings>
<add name="con" connectionString="Data
Source=localhost; Initial Catalog= Blog; Integrated Security=true;" providerName="System.Data.SqlClient"/>
</connectionStrings>
ASP.NET Design Section:
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<fieldset style="width:280px"><legend><strong> Bind DropdownList
and Set Default</strong></legend>
<div>
<asp:DropDownList ID="ddlstudent" runat="server"></asp:DropDownList>
</div>
</fieldset>
</form>
</body>
</html>
ASP.NET code behind File using C#:
Add following
Namespaces:
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
C# Code:
public partial class Dropdown : System.Web.UI.Page
{
SqlConnection con = new SqlConnection(ConfigurationManager.ConnectionStrings["con"].ConnectionString);
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
Fill_DropDown();
}
}
//Fetch data from
database and bind to gridview
public void Fill_DropDown()
{
if (con.State == ConnectionState.Open)
{
con.Close();
}
con.Open();
DataSet ds = new DataSet();
SqlCommand cmd = new SqlCommand();
cmd.Connection = con;
cmd.CommandText = "Fill_Dataset";
cmd.CommandType = CommandType.StoredProcedure;
SqlDataAdapter dataadapater = new SqlDataAdapter();
dataadapater.SelectCommand = cmd;
dataadapater.Fill(ds);
ddlstudent.DataSource = ds;
ddlstudent.DataTextField = "Student_name";
ddlstudent.DataValueField = "Student_id";
ddlstudent.DataBind();
ddlstudent.Items.Insert(0,"Select Student");
}
}
Vb.Net Code:
Add
following namespaces:
Imports System.Data
Imports System.Data.SqlClient
Imports System.Configuration
Now
Write Following Code:
Partial Public Class Dropdown
Inherits System.Web.UI.Page
Private con As New SqlConnection(ConfigurationManager.ConnectionStrings("con").ConnectionString)
Protected Sub Page_Load(sender As Object, e As EventArgs)
If Not IsPostBack Then
Fill_DropDown()
End If
End Sub
'Fetch data from
database and bind to gridview
Public Sub Fill_DropDown()
If con.State =
ConnectionState.Open Then
con.Close()
End If
con.Open()
Dim ds As New DataSet()
Dim cmd As New SqlCommand()
cmd.Connection = con
cmd.CommandText = "Fill_Dataset"
cmd.CommandType = CommandType.StoredProcedure
Dim dataadapater As New SqlDataAdapter()
dataadapater.SelectCommand = cmd
dataadapater.Fill(ds)
ddlstudent.DataSource = ds
ddlstudent.DataTextField = "Student_name"
ddlstudent.DataValueField = "Student_id"
ddlstudent.DataBind()
ddlstudent.Items.Insert(0, "Select Student")
End Sub
End Class
0 comments:
Post a Comment